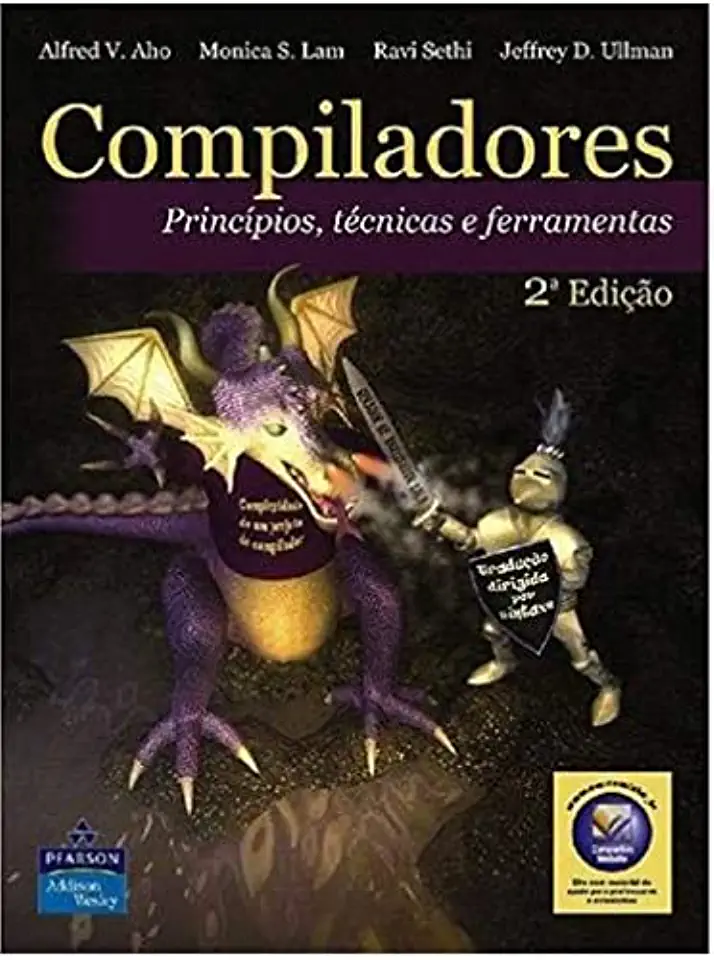
Compilers- Principles, Techniques, and Tools - Alfred V. Aho, Ravi Sethi, Jeffrey D. Ullman
Compilers: Principles, Techniques, and Tools
Introduction
Compilers are essential tools for software development. They translate high-level programming languages into machine code that can be executed by computers. This book provides a comprehensive introduction to the principles, techniques, and tools used in compiler design and implementation.
Key Features
- Comprehensive coverage: This book covers all aspects of compiler design and implementation, from lexical analysis and parsing to code generation and optimization.
- Clear and concise explanations: The material is presented in a clear and concise manner, making it easy for readers to understand even complex concepts.
- Numerous examples: The book includes numerous examples to illustrate the concepts discussed. These examples help readers to understand how compilers work and how to apply the techniques they learn.
- Exercises: Each chapter includes exercises that help readers to test their understanding of the material.
- Solutions manual: A solutions manual is available for instructors who adopt the book for their courses.
Benefits of Reading This Book
This book is essential reading for anyone who wants to learn about compiler design and implementation. It is also a valuable resource for software developers who want to understand how compilers work and how to write code that is efficient and easy to compile.
Conclusion
Compilers are essential tools for software development. This book provides a comprehensive introduction to the principles, techniques, and tools used in compiler design and implementation. It is a valuable resource for anyone who wants to learn about compilers or write better code.
Table of Contents
- Part I: Introduction
- Chapter 1: Introduction to Compilers
- Chapter 2: The Structure of a Compiler
- Part II: Lexical Analysis
- Chapter 3: Lexical Analysis
- Chapter 4: Regular Expressions
- Chapter 5: Finite Automata
- Part III: Syntax Analysis
- Chapter 6: Context-Free Grammars
- Chapter 7: Top-Down Parsing
- Chapter 8: Bottom-Up Parsing
- Part IV: Semantic Analysis
- Chapter 9: Semantic Analysis
- Chapter 10: Type Checking
- Chapter 11: Symbol Tables
- Part V: Intermediate Code Generation
- Chapter 12: Intermediate Code Generation
- Chapter 13: Three-Address Code
- Chapter 14: Control Flow Graphs
- Part VI: Code Optimization
- Chapter 15: Code Optimization
- Chapter 16: Local Optimization
- Chapter 17: Global Optimization
- Part VII: Code Generation
- Chapter 18: Code Generation
- Chapter 19: Machine-Independent Code Generation
- Chapter 20: Machine-Dependent Code Generation
About the Authors
Alfred V. Aho is a Distinguished Professor of Computer Science at Columbia University. He is a member of the National Academy of Engineering and a Fellow of the American Academy of Arts and Sciences. He is the author of numerous books and articles on compiler design, algorithms, and data structures.
Ravi Sethi is a Professor of Computer Science at the University of California, Santa Cruz. He is a Fellow of the American Association for Artificial Intelligence and a member of the Association for Computing Machinery. He is the author of numerous books and articles on compiler design, artificial intelligence, and natural language processing.
Jeffrey D. Ullman is a Professor of Computer Science at Stanford University. He is a member of the National Academy of Engineering and a Fellow of the American Academy of Arts and Sciences. He is the author of numerous books and articles on compiler design, databases, and algorithms.
Enjoyed the summary? Discover all the details and take your reading to the next level — [click here to view the book on Amazon!]