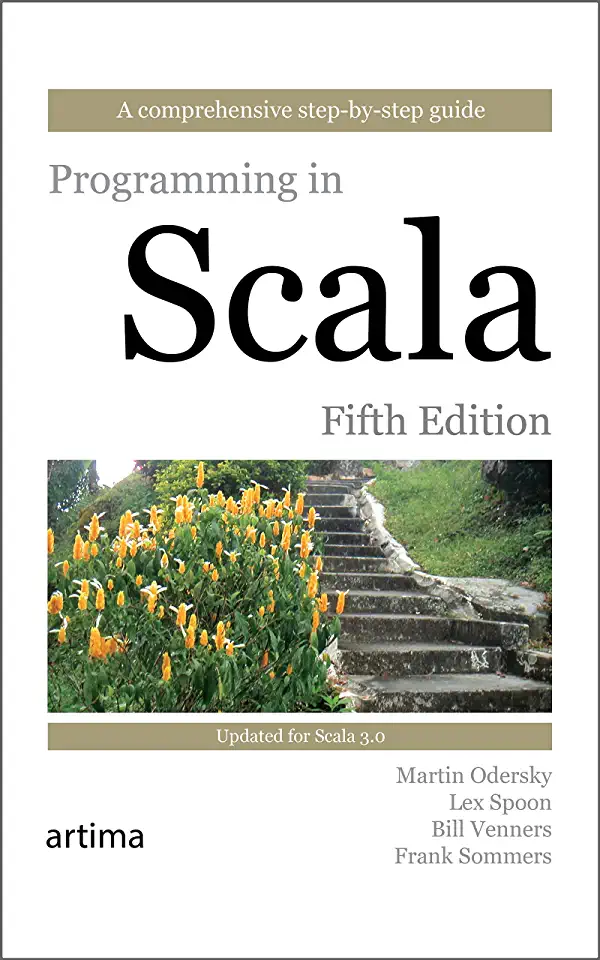
Programming in Scala- Updated for Scala 2.12 - Martin Odersky, Lex Spoon, Bill Venners
Programming in Scala: Updated for Scala 2.12
A Comprehensive Guide to Scala Programming
Programming in Scala is the definitive guide to Scala, the powerful and versatile programming language that combines object-oriented and functional programming paradigms. Written by the language's creators, Martin Odersky, Lex Spoon, and Bill Venners, this book provides a comprehensive introduction to Scala, from its basic syntax to its advanced features.
Why Scala?
Scala is a modern, general-purpose programming language that offers a number of advantages over other languages, including:
- Expressiveness: Scala's concise syntax and powerful features make it easy to write code that is both readable and maintainable.
- Scalability: Scala is designed to support large-scale applications, with features such as type inference and immutability that help to prevent errors and improve performance.
- Concurrency: Scala's support for concurrency makes it easy to write code that can take advantage of multiple processors or cores.
- Interoperability: Scala is interoperable with Java, allowing you to reuse existing Java code and libraries.
What You'll Learn
Programming in Scala will teach you everything you need to know to get started with Scala, including:
- The basics of Scala syntax, including variables, types, and expressions
- Object-oriented programming in Scala, including classes, traits, and inheritance
- Functional programming in Scala, including functions, closures, and higher-order functions
- Concurrency in Scala, including threads, locks, and futures
- Interoperability with Java, including how to use Scala code in Java applications
Who This Book Is For
Programming in Scala is ideal for anyone who wants to learn Scala, from beginners to experienced programmers. Whether you're looking to build web applications, mobile apps, or distributed systems, Scala is a powerful tool that can help you get the job done.
Get Started with Scala Today
Programming in Scala is the essential guide to Scala, the powerful and versatile programming language that is taking the world by storm. Get your copy today and start learning Scala!
Table of Contents
- Part I: Getting Started
- Chapter 1: Introduction to Scala
- Chapter 2: Basic Syntax
- Chapter 3: Types and Type Inference
- Chapter 4: Expressions and Control Structures
- Part II: Object-Oriented Programming
- Chapter 5: Classes and Objects
- Chapter 6: Traits
- Chapter 7: Inheritance
- Chapter 8: Polymorphism
- Part III: Functional Programming
- Chapter 9: Functions
- Chapter 10: Closures
- Chapter 11: Higher-Order Functions
- Chapter 12: Pattern Matching
- Part IV: Concurrency
- Chapter 13: Threads
- Chapter 14: Locks
- Chapter 15: Futures
- Part V: Interoperability with Java
- Chapter 16: Using Scala Code in Java Applications
- Chapter 17: Using Java Libraries in Scala Applications
- Part VI: Advanced Topics
- Chapter 18: Macros
- Chapter 19: Reflection
- Chapter 20: Scala Native
Appendixes
- Appendix A: Scala Language Specification
- Appendix B: Scala Standard Library
- Appendix C: Scala Tools
- Appendix D: Scala Community
Index
Enjoyed the summary? Discover all the details and take your reading to the next level — [click here to view the book on Amazon!]